This small script monitors mouse movement and preloads content that a user is likely to click on.
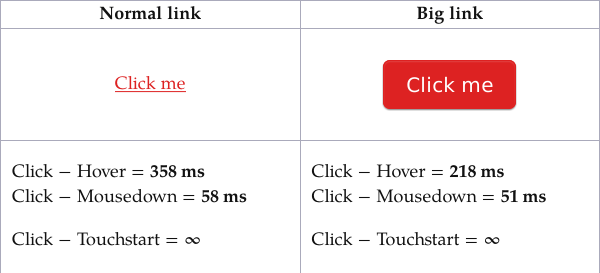
Results from http://instantclick.io/click-test
This means that between hover and click there were between 260-400 unused milliseconds which could have been used to fetch the HTML content.
This simple script aims to utilize that time buy fetching the HTML content and preventing the click action with an event that replaces the current page content.
//var is used for older device support but can be replaced with let if this is not a concern
var pagesStore = [];
var pagesQueued = [];
document.addEventListener("mouseover", function (event) {
if (typeof event.target.href != "undefined") {
console.log(event.target.href);
fetchURL(event.target.href)
} else {
if (typeof event.target.parentElement != "undefined" || event.target.parentElement != null) {
if (typeof event.target.parentElement.href != "undefined") {
console.log(event.target.parentElement.href);
fetchURL(event.target.parentElement.href)
}
}
}
}, false);
document.addEventListener("click", function (event) {
if (typeof event.target.href != "undefined") {
console.log(event.target.href);
loadFetchedPage(event.target.href)
} else {
if (typeof event.target.parentElement != "undefined" || event.target.parentElement != null) {
if (typeof event.target.parentElement.href != "undefined") {
console.log(event.target.parentElement.href);
loadFetchedPage(event.target.parentElement.href)
}
}
}
}, false);
function fetchURL(urlToFetch) {
if (typeof pagesQueued[urlToFetch] == "undefined") {
pagesQueued[urlToFetch] = true;
console.log("Fetching " + urlToFetch);
console.log(this);
var xmlhttp;
if (window.XMLHttpRequest) {
xmlhttp = new XMLHttpRequest();
} else { // code for IE6, IE5
xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttp.onreadystatechange = function () {
if (xmlhttp.readyState === 4 && xmlhttp.status === 200) {
pagesStore[urlToFetch] = xmlhttp.responseText;
}
};
xmlhttp.open("GET", urlToFetch, true);
xmlhttp.send();
}
}
function loadFetchedPage(urlToLoad) {
if (typeof pagesStore[urlToLoad] == "undefined") {
//console.log('Loading ' + urlToLoad);
//window.location = urlToLoad;
} else {
event.preventDefault();
history.pushState('', urlToLoad, urlToLoad);
console.log('Using cached ' + urlToLoad);
document.open();
document.write(pagesStore[urlToLoad]);
document.close();
}
}